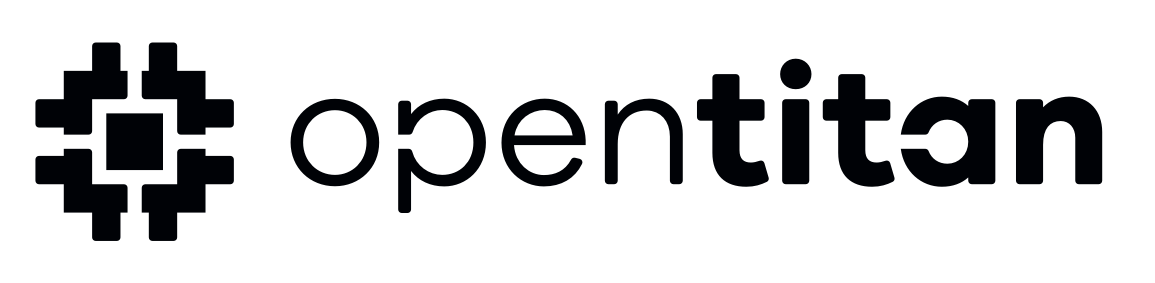 |
Software APIs
|
4 #ifndef OPENTITAN_SW_HOST_TESTS_USBDEV_USBDEV_STREAM_STREAM_TEST_H_
5 #define OPENTITAN_SW_HOST_TESTS_USBDEV_USBDEV_STREAM_STREAM_TEST_H_
10 #ifdef USBDEV_NUM_ENDPOINTS
11 #define STREAMS_MAX (USBDEV_NUM_ENDPOINTS - 1U)
13 #define STREAMS_MAX 11U
23 TestConfig(
bool init_verb,
bool init_retr,
bool init_chk,
bool init_send)
69 extern uint64_t start_time;
77 void ReportSyntax(
void);