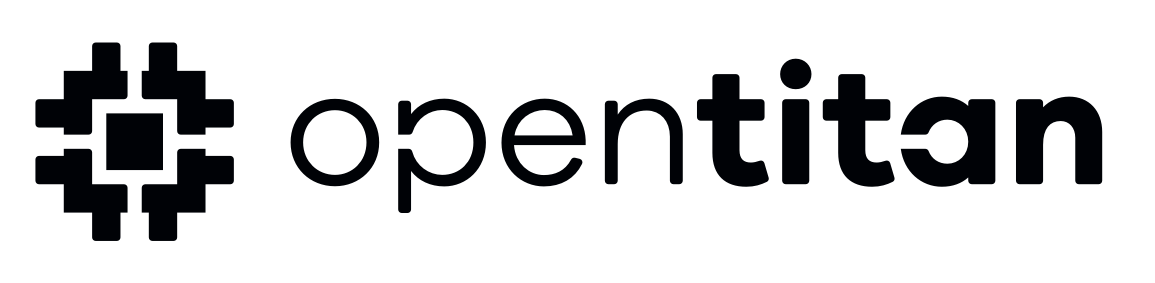 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_OWNERSHIP_ECDSA_H_
6 #define OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_OWNERSHIP_ECDSA_H_
11 #include "sw/device/silicon_creator/lib/drivers/hmac.h"
12 #include "sw/device/silicon_creator/lib/error.h"
13 #include "sw/device/silicon_creator/lib/ownership/datatypes.h"
22 rom_error_t ecdsa_init(
void);
52 const void *message,
size_t message_len);