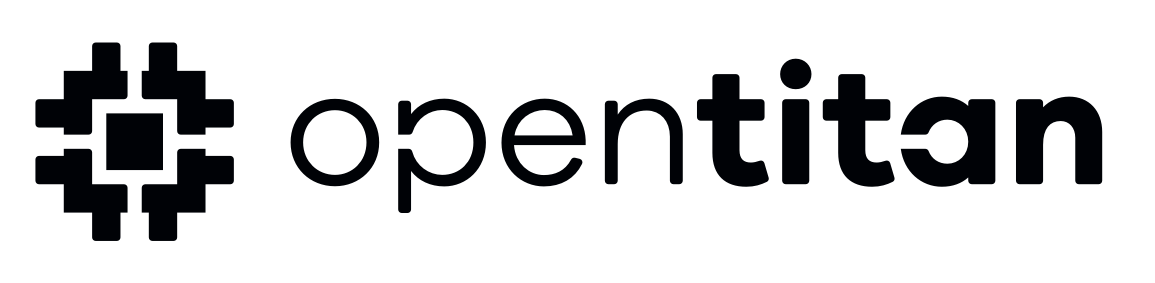 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_LIB_TESTING_TEST_FRAMEWORK_OTTF_TEST_CONFIG_H_
6 #define OPENTITAN_SW_DEVICE_LIB_TESTING_TEST_FRAMEWORK_OTTF_TEST_CONFIG_H_
11 typedef enum ottf_console_type {
13 kOttfConsoleSpiDevice,
14 } ottf_console_type_t;
85 bool silence_console_prints;
110 #define OTTF_DEFINE_TEST_CONFIG(...) \
111 const ottf_test_config_t kOttfTestConfig = {.file = __FILE__, __VA_ARGS__}