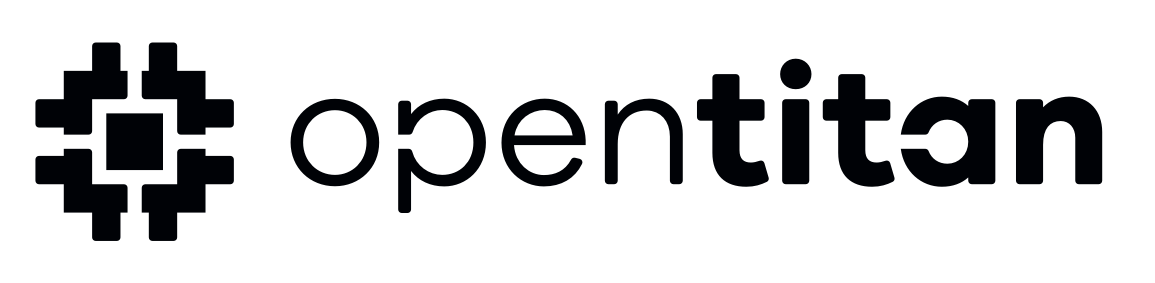 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_SILICON_CREATOR_MANUF_LIB_OTP_IMG_TYPES_H_
6 #define OPENTITAN_SW_DEVICE_SILICON_CREATOR_MANUF_LIB_OTP_IMG_TYPES_H_
17 typedef enum otp_val_type {
19 kOptValTypeUint32Buff,
21 kOptValTypeUint64Buff,
49 const uint32_t *value32;
54 const uint64_t *value64;