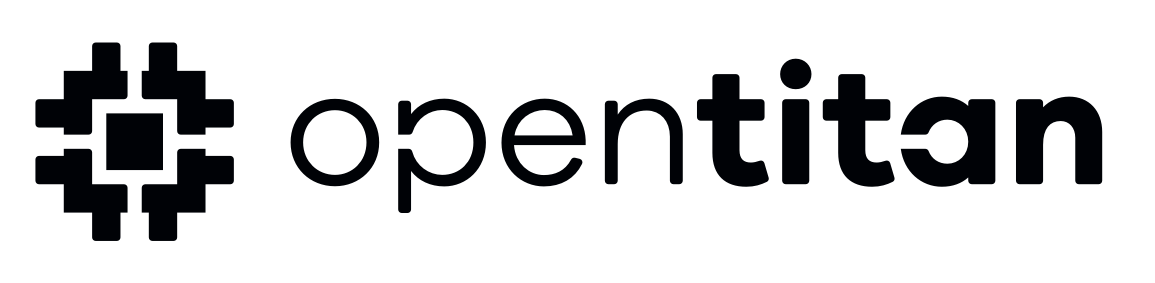 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_LIB_TESTING_HEXSTR_H_
6 #define OPENTITAN_SW_DEVICE_LIB_TESTING_HEXSTR_H_
10 #include "sw/device/lib/base/status.h"
26 status_t hexstr_encode(
char *dst,
size_t dst_size,
const void *src,
37 status_t hexstr_decode(
void *dst,
size_t dst_size,
const char *src);