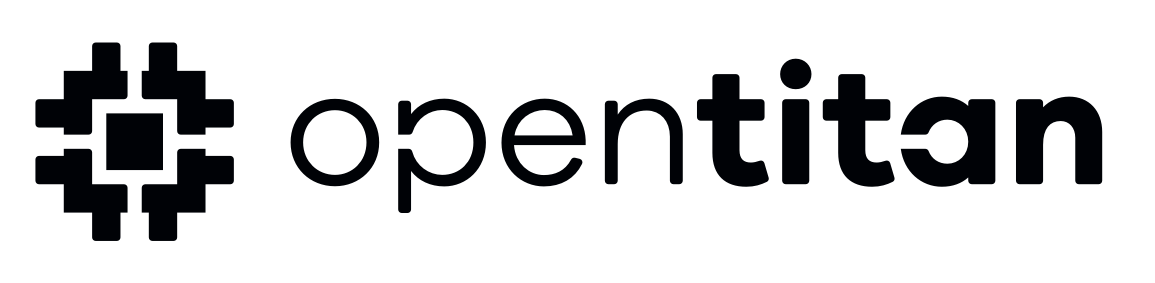 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_EPMP_DEFS_H_
6 #define OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_EPMP_DEFS_H_
26 #define EPMP_MSECCFG 0x747
35 #define EPMP_MSECCFG_MML (1 << 0)
36 #define EPMP_MSECCFG_MMWP (1 << 1)
37 #define EPMP_MSECCFG_RLB (1 << 2)
58 #define EPMP_CFG_L (1 << 7)
59 #define EPMP_CFG_A_MASK (3 << 3)
60 #define EPMP_CFG_A_OFF (0 << 3)
61 #define EPMP_CFG_A_TOR (1 << 3)
62 #define EPMP_CFG_A_NA4 (2 << 3)
63 #define EPMP_CFG_A_NAPOT (3 << 3)
64 #define EPMP_CFG_X (1 << 2)
65 #define EPMP_CFG_W (1 << 1)
66 #define EPMP_CFG_R (1 << 0)
71 #define EPMP_CFG_LR (EPMP_CFG_L | EPMP_CFG_R)
72 #define EPMP_CFG_LRX (EPMP_CFG_L | EPMP_CFG_R | EPMP_CFG_X)
73 #define EPMP_CFG_LRW (EPMP_CFG_L | EPMP_CFG_R | EPMP_CFG_W)
74 #define EPMP_CFG_LRWX (EPMP_CFG_L | EPMP_CFG_R | EPMP_CFG_W | EPMP_CFG_X)