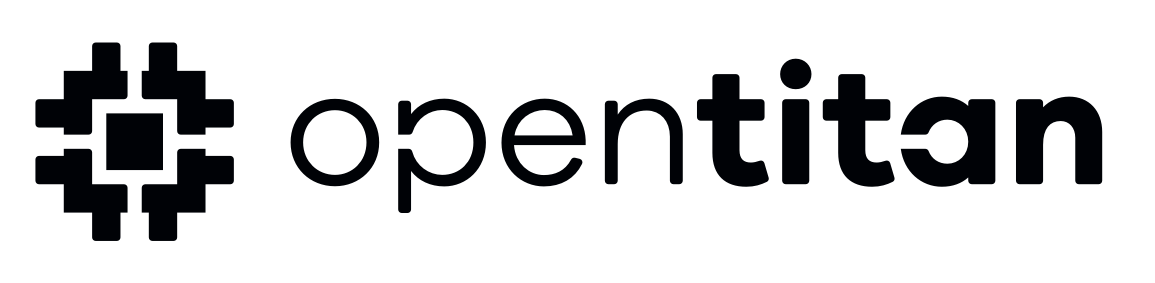 |
Software APIs
|
Go to the documentation of this file.
7#ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_SOC_PROXY_AUTOGEN_H_
8#define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_SOC_PROXY_AUTOGEN_H_
247#define kDifSocProxyIrqExternal0 kDtSocProxyIrqExternal0
248#define kDifSocProxyIrqExternal1 kDtSocProxyIrqExternal1
249#define kDifSocProxyIrqExternal2 kDtSocProxyIrqExternal2
250#define kDifSocProxyIrqExternal3 kDtSocProxyIrqExternal3
251#define kDifSocProxyIrqExternal4 kDtSocProxyIrqExternal4
252#define kDifSocProxyIrqExternal5 kDtSocProxyIrqExternal5
253#define kDifSocProxyIrqExternal6 kDtSocProxyIrqExternal6
254#define kDifSocProxyIrqExternal7 kDtSocProxyIrqExternal7
255#define kDifSocProxyIrqExternal8 kDtSocProxyIrqExternal8
256#define kDifSocProxyIrqExternal9 kDtSocProxyIrqExternal9
257#define kDifSocProxyIrqExternal10 kDtSocProxyIrqExternal10
258#define kDifSocProxyIrqExternal11 kDtSocProxyIrqExternal11
259#define kDifSocProxyIrqExternal12 kDtSocProxyIrqExternal12
260#define kDifSocProxyIrqExternal13 kDtSocProxyIrqExternal13
261#define kDifSocProxyIrqExternal14 kDtSocProxyIrqExternal14
262#define kDifSocProxyIrqExternal15 kDtSocProxyIrqExternal15
263#define kDifSocProxyIrqExternal16 kDtSocProxyIrqExternal16
264#define kDifSocProxyIrqExternal17 kDtSocProxyIrqExternal17
265#define kDifSocProxyIrqExternal18 kDtSocProxyIrqExternal18
266#define kDifSocProxyIrqExternal19 kDtSocProxyIrqExternal19
267#define kDifSocProxyIrqExternal20 kDtSocProxyIrqExternal20
268#define kDifSocProxyIrqExternal21 kDtSocProxyIrqExternal21
269#define kDifSocProxyIrqExternal22 kDtSocProxyIrqExternal22
270#define kDifSocProxyIrqExternal23 kDtSocProxyIrqExternal23
271#define kDifSocProxyIrqExternal24 kDtSocProxyIrqExternal24
272#define kDifSocProxyIrqExternal25 kDtSocProxyIrqExternal25
273#define kDifSocProxyIrqExternal26 kDtSocProxyIrqExternal26
274#define kDifSocProxyIrqExternal27 kDtSocProxyIrqExternal27
275#define kDifSocProxyIrqExternal28 kDtSocProxyIrqExternal28
276#define kDifSocProxyIrqExternal29 kDtSocProxyIrqExternal29
277#define kDifSocProxyIrqExternal30 kDtSocProxyIrqExternal30
278#define kDifSocProxyIrqExternal31 kDtSocProxyIrqExternal31
364 dif_soc_proxy_irq_t);