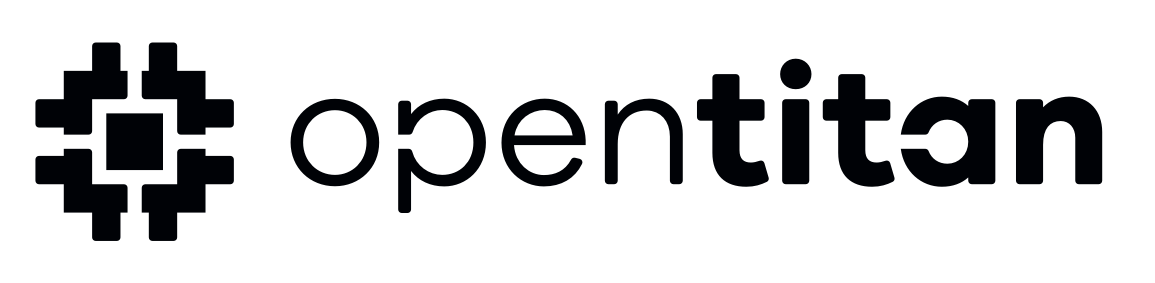 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_PWRMGR_AUTOGEN_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_PWRMGR_AUTOGEN_H_
236 #endif // __cplusplus
238 #endif // OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_PWRMGR_AUTOGEN_H_