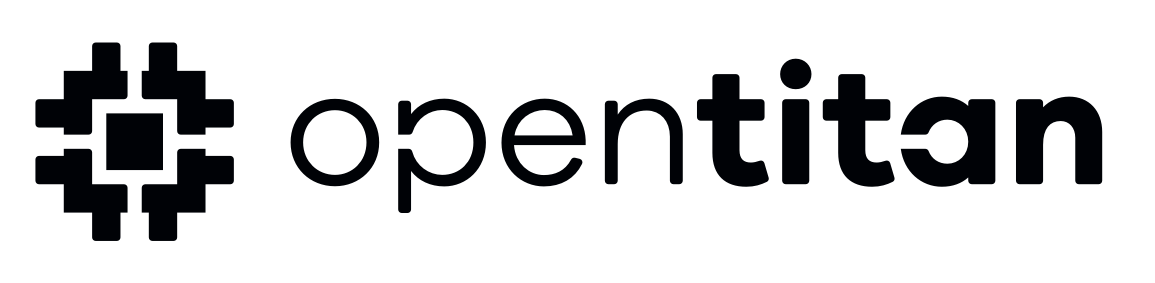 |
Software APIs
|
Go to the documentation of this file.
5#ifndef OPENTITAN_SW_DEVICE_LIB_DIF_DIF_PWM_H_
6#define OPENTITAN_SW_DEVICE_LIB_DIF_DIF_PWM_H_
46#define DIF_PWM_CHANNEL_LIST(X) \
58#define PWM_CHANNEL_ENUM_INIT_(channel_) \
59 kDifPwmChannel##channel_ = 1U << channel_,
68#undef PWM_CHANNEL_ENUM_INIT_