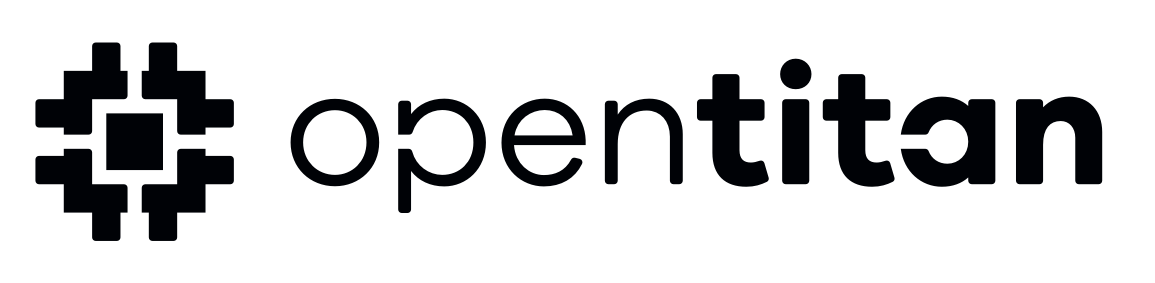 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_DIF_PATTGEN_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_DIF_PATTGEN_H_
18 #include "sw/device/lib/dif/autogen/dif_pattgen_autogen.h"
29 #define DIF_PATTGEN_CHANNEL_LIST(X) \
37 #define PATTGEN_CHANNEL_ENUM_INIT_(channel_) \
38 kDifPattgenChannel##channel_ = channel_,
47 #undef PATTGEN_CHANNEL_ENUM_INIT_