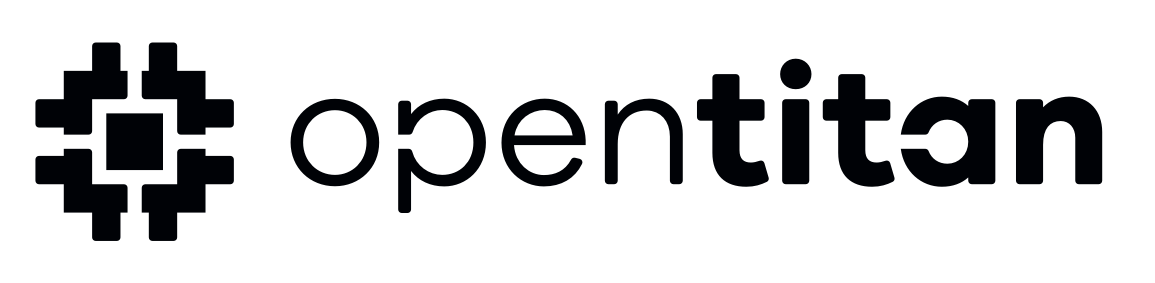 |
Software APIs
|
Go to the documentation of this file.
7#ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_MBX_AUTOGEN_H_
8#define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_MBX_AUTOGEN_H_
138#define kDifMbxIrqMbxReady kDtMbxIrqMbxReady
142#define kDifMbxIrqMbxAbort kDtMbxIrqMbxAbort
146#define kDifMbxIrqMbxError kDtMbxIrqMbxError