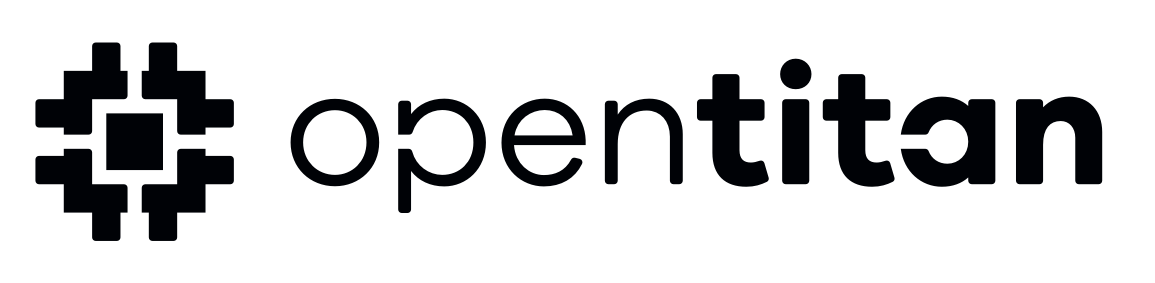 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_I2C_AUTOGEN_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_I2C_AUTOGEN_H_
304 #endif // __cplusplus
306 #endif // OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_I2C_AUTOGEN_H_