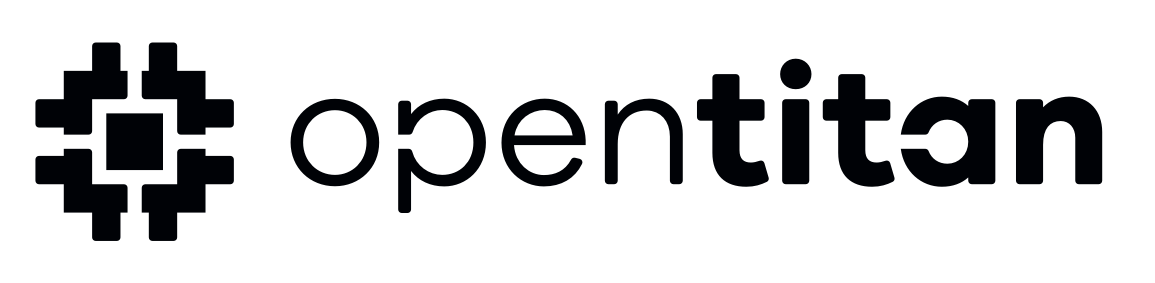 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_GPIO_AUTOGEN_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_GPIO_AUTOGEN_H_
91 kDifGpioIrqGpio10 = 10,
92 kDifGpioIrqGpio11 = 11,
93 kDifGpioIrqGpio12 = 12,
94 kDifGpioIrqGpio13 = 13,
95 kDifGpioIrqGpio14 = 14,
96 kDifGpioIrqGpio15 = 15,
97 kDifGpioIrqGpio16 = 16,
98 kDifGpioIrqGpio17 = 17,
99 kDifGpioIrqGpio18 = 18,
100 kDifGpioIrqGpio19 = 19,
101 kDifGpioIrqGpio20 = 20,
102 kDifGpioIrqGpio21 = 21,
103 kDifGpioIrqGpio22 = 22,
104 kDifGpioIrqGpio23 = 23,
105 kDifGpioIrqGpio24 = 24,
106 kDifGpioIrqGpio25 = 25,
107 kDifGpioIrqGpio26 = 26,
108 kDifGpioIrqGpio27 = 27,
109 kDifGpioIrqGpio28 = 28,
110 kDifGpioIrqGpio29 = 29,
111 kDifGpioIrqGpio30 = 30,
112 kDifGpioIrqGpio31 = 31,
263 #endif // __cplusplus
265 #endif // OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_GPIO_AUTOGEN_H_