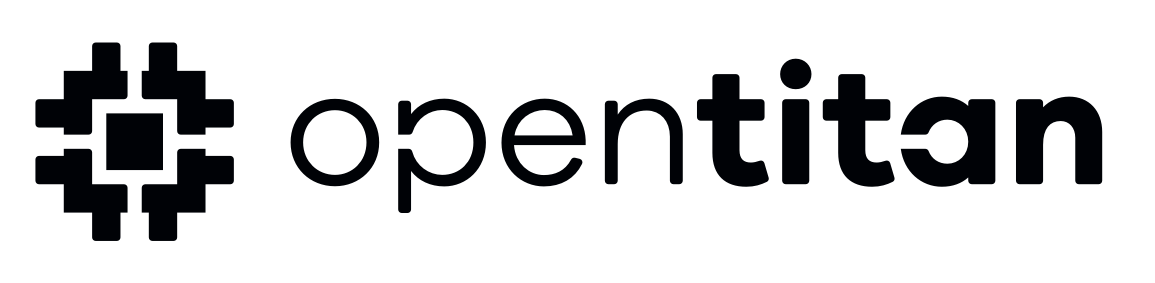 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_FLASH_CTRL_AUTOGEN_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_FLASH_CTRL_AUTOGEN_H_
287 #endif // __cplusplus
289 #endif // OPENTITAN_SW_DEVICE_LIB_DIF_AUTOGEN_DIF_FLASH_CTRL_AUTOGEN_H_