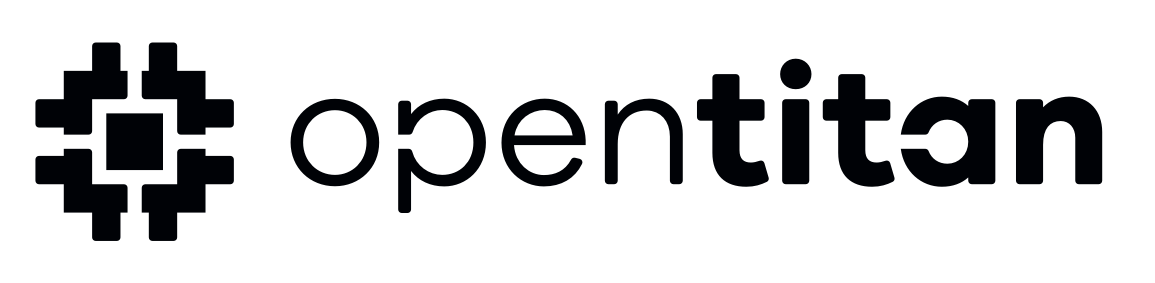 |
Software APIs
|
Go to the documentation of this file.
5 #ifndef OPENTITAN_SW_DEVICE_LIB_DIF_DIF_BASE_H_
6 #define OPENTITAN_SW_DEVICE_LIB_DIF_DIF_BASE_H_
16 #include "sw/device/lib/base/multibits.h"
18 #define USING_INTERNAL_STATUS
19 #include "sw/device/lib/base/internal/status.h"
20 #undef USING_INTERNAL_STATUS
31 #define DIF_RETURN_IF_ERROR(expr_) \
33 dif_result_t local_error_ = (expr_); \
34 if (local_error_ != kDifOk) { \
35 return local_error_; \
183 case kMultiBitBool4True:
184 case kMultiBitBool8True:
185 case kMultiBitBool12True:
186 case kMultiBitBool16True:
203 return kMultiBitBool4True;
205 return kMultiBitBool4False;
219 return kMultiBitBool8True;
221 return kMultiBitBool8False;
235 return kMultiBitBool12True;
237 return kMultiBitBool12False;
251 return kMultiBitBool16True;
253 return kMultiBitBool16False;
259 #endif // __cplusplus
261 #endif // OPENTITAN_SW_DEVICE_LIB_DIF_DIF_BASE_H_