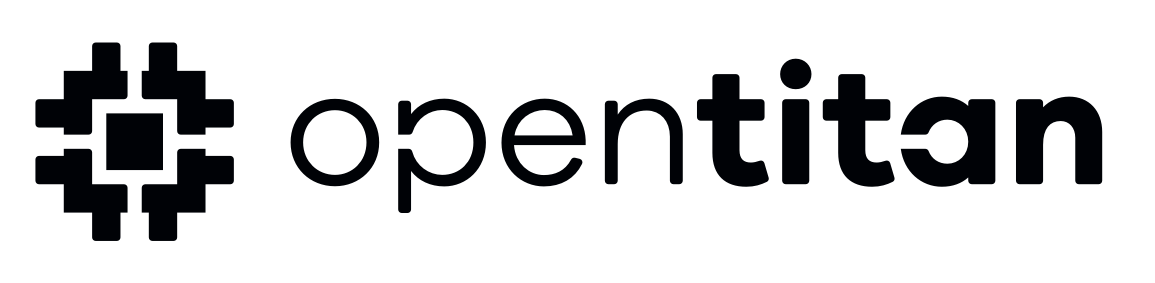 |
Software APIs
|
Go to the documentation of this file.
5#ifndef OPENTITAN_SW_DEVICE_LIB_ARCH_DEVICE_H_
6#define OPENTITAN_SW_DEVICE_LIB_ARCH_DEVICE_H_
131#define CALCULATE_UART_NCO_(baudrate, peripheral_clock) \
132 (baudrate == 1500000 && peripheral_clock == 24000000) \
134 : (uint32_t)(((uint64_t)(baudrate) << (16 + 4)) / (peripheral_clock))
136#define CALCULATE_UART_NCO(baudrate, peripheral_clock) \
137 CALCULATE_UART_NCO_(baudrate, peripheral_clock) < 0x10000 \
138 ? CALCULATE_UART_NCO_(baudrate, peripheral_clock) \
151extern const uint32_t kUartBaud230K;
152extern const uint32_t kUartBaud460K;
153extern const uint32_t kUartBaud921K;
154extern const uint32_t kUartBaud1M33;
155extern const uint32_t kUartBaud1M50;
164#define CALCULATE_UART_TX_FIFO_CPU_CYCLES(baud_rate_, cpu_freq_, fifo_depth_) \
165 ((cpu_freq_)*10 * (fifo_depth_) / (baud_rate_))
178#define CALCULATE_AST_CHECK_POLL_CPU_CYCLES(cpu_freq_) \
179 ((cpu_freq_)*100 / 1000000)