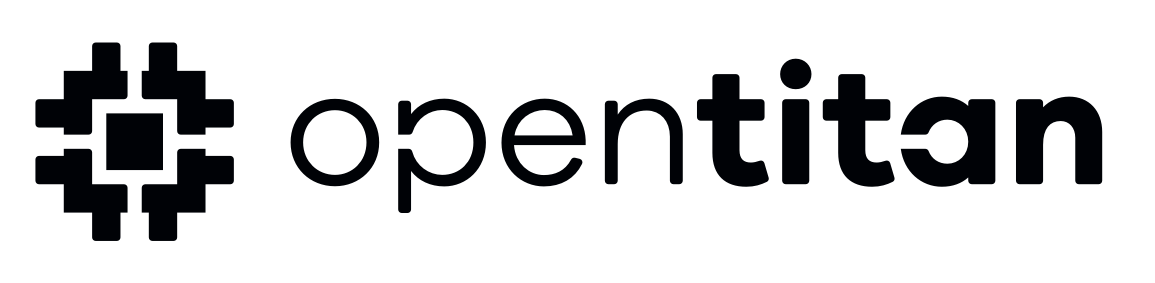 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_LIB_CRYPTO_IMPL_STATUS_H_
6 #define OPENTITAN_SW_DEVICE_LIB_CRYPTO_IMPL_STATUS_H_
9 #include "sw/device/lib/base/status.h"
28 #define OTCRYPTO_OK ((status_t){.value = kHardenedBoolTrue})
29 #ifdef OTCRYPTO_STATUS_DEBUG
31 #define OTCRYPTO_RECOV_ERR \
32 ((status_t){.value = (int32_t)(0x80000000 | MODULE_ID | \
33 ((__LINE__ & 0x7ff) << 5) | kAborted)})
34 #define OTCRYPTO_FATAL_ERR \
35 ((status_t){.value = \
36 (int32_t)(0x80000000 | MODULE_ID | \
37 ((__LINE__ & 0x7ff) << 5) | kFailedPrecondition)})
38 #define OTCRYPTO_BAD_ARGS \
39 ((status_t){.value = \
40 (int32_t)(0x80000000 | MODULE_ID | \
41 ((__LINE__ & 0x7ff) << 5) | kInvalidArgument)})
42 #define OTCRYPTO_ASYNC_INCOMPLETE \
43 ((status_t){.value = (int32_t)(0x80000000 | MODULE_ID | \
44 ((__LINE__ & 0x7ff) << 5) | kUnavailable)})
45 #define OTCRYPTO_NOT_IMPLEMENTED \
46 ((status_t){.value = (int32_t)(0x80000000 | MODULE_ID | \
47 ((__LINE__ & 0x7ff) << 5) | kUnimplemented)})
50 #define OTCRYPTO_RECOV_ERR \
51 ((status_t){.value = kOtcryptoStatusValueInternalError})
52 #define OTCRYPTO_FATAL_ERR ((status_t){.value = kOtcryptoStatusValueFatalError})
53 #define OTCRYPTO_BAD_ARGS ((status_t){.value = kOtcryptoStatusValueBadArgs})
54 #define OTCRYPTO_ASYNC_INCOMPLETE \
55 ((status_t){.value = kOtcryptoStatusValueAsyncIncomplete})
56 #define OTCRYPTO_NOT_IMPLEMENTED \
57 ((status_t){.value = kOtcryptoStatusValueNotImplemented})
70 #define HARDENED_TRY(expr_) \
72 status_t status_ = expr_; \
73 if (!status_ok(status_)) { \
76 if (launder32(OT_UNSIGNED(status_.value)) != kHardenedBoolTrue) { \
77 return OTCRYPTO_FATAL_ERR; \
79 HARDENED_CHECK_EQ(status_.value, kHardenedBoolTrue); \