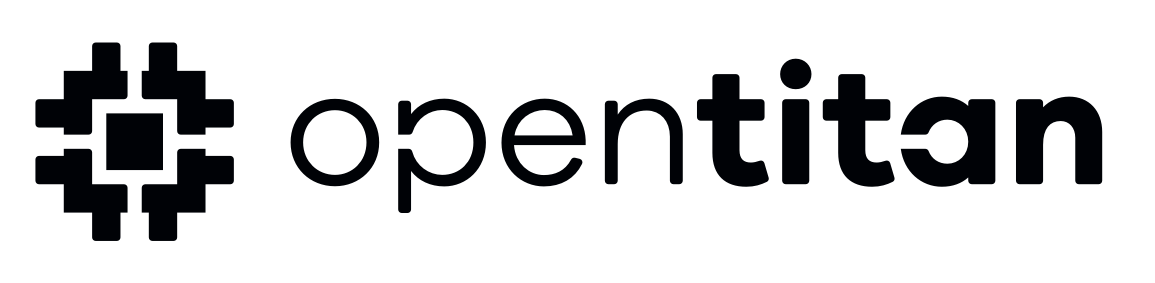 |
Software APIs
|
Go to the documentation of this file.
5#ifndef OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_BASE_CHIP_H_
6#define OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_BASE_CHIP_H_
16#define CHIP_MANIFEST_SIZE 1024
21#define CHIP_MANIFEST_VERSION_MINOR_1 0x6c47
22#define CHIP_MANIFEST_VERSION_MAJOR_1 0x71c3
26#define CHIP_MANIFEST_VERSION_MAJOR_2 0x0002
31#define CHIP_MANIFEST_EXT_TABLE_ENTRY_COUNT 15
36#define CHIP_BOOT_SVC_MSG_HEADER_SIZE 44
41#define CHIP_BOOT_SVC_MSG_SIZE_MAX 256
46#define CHIP_BOOT_SVC_MSG_PAYLOAD_SIZE_MAX \
47 (CHIP_BOOT_SVC_MSG_SIZE_MAX - CHIP_BOOT_SVC_MSG_HEADER_SIZE)
52#define CHIP_BL0_IDENTIFIER 0x3042544f
57#define CHIP_BL0_SIZE_MIN CHIP_MANIFEST_SIZE
58#define CHIP_BL0_SIZE_MAX 0x70000
63#define CHIP_ROM_EXT_IDENTIFIER 0x4552544f
68#define CHIP_ROM_EXT_SIZE_MIN CHIP_MANIFEST_SIZE
69#define CHIP_ROM_EXT_SIZE_MAX 0x10000
70#define CHIP_ROM_EXT_RESIZABLE_SIZE_MAX \
71 (CHIP_ROM_EXT_SIZE_MAX + CHIP_BL0_SIZE_MAX)
77#define TEST_ROM_IDENTIFIER 0x54534554
86#define PINMUX_PAD_ATTR_PROP_CYCLES 500
91#define SW_STRAP_0_PERIPH 22
92#define SW_STRAP_1_PERIPH 23
93#define SW_STRAP_2_PERIPH 24
98#define SW_STRAP_0_INSEL 24
99#define SW_STRAP_1_INSEL 25
100#define SW_STRAP_2_INSEL 26
105#define SW_STRAP_0_PAD 22
106#define SW_STRAP_1_PAD 23
107#define SW_STRAP_2_PAD 24
112#define SW_STRAP_MASK \
113 ((1 << SW_STRAP_2_PERIPH) | (1 << SW_STRAP_1_PERIPH) | \
114 (1 << SW_STRAP_0_PERIPH))
122#define SW_STRAP_RMA_ENTRY \
123 ((1 << SW_STRAP_2_PERIPH) | (1 << SW_STRAP_1_PERIPH) | \
124 (0 << SW_STRAP_0_PERIPH))
131#define SW_STRAP_BOOTSTRAP \
132 ((1 << SW_STRAP_2_PERIPH) | (1 << SW_STRAP_1_PERIPH) | \
133 (1 << SW_STRAP_0_PERIPH))