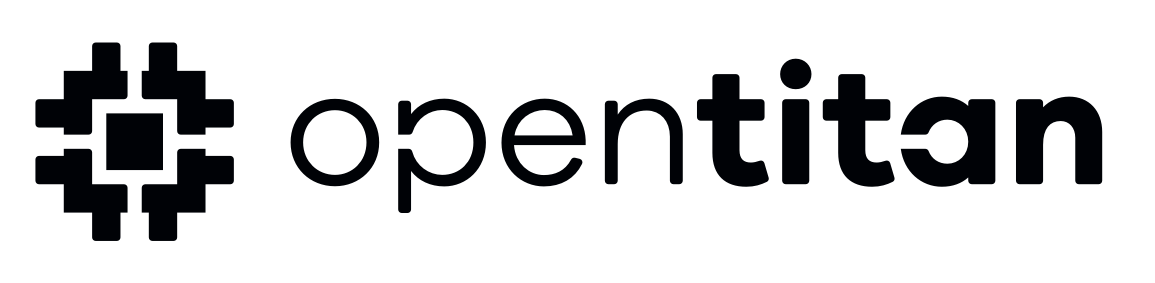 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_CFI_H_
6 #define OPENTITAN_SW_DEVICE_SILICON_CREATOR_LIB_CFI_H_
77 #define CFI_FUNC_COUNTER_INIT_CONSTANTS_(name_, value_) name_##Val0 = value_,
82 #define CFI_FUNC_COUNTERS_TABLE_INIT_(name_, value_) 0,
87 #define CFI_FUNC_COUNTER_INDEXES_(name_, value_) name_,
97 #define CFI_DEFINE_COUNTERS(table_name_, table_) \
98 enum { table_(CFI_FUNC_COUNTER_INIT_CONSTANTS_) }; \
99 enum { table_(CFI_FUNC_COUNTER_INDEXES_) }; \
100 uint32_t table_name_[] = {table_(CFI_FUNC_COUNTERS_TABLE_INIT_)}
105 kCfiIncrement = 0x5a,
117 #define CFI_FUNC_COUNTER_INIT(table, index) \
119 table[index] = (index##Val0 + kCfiIncrement); \
120 barrier32(table[index]); \
129 #define CFI_STEP_TO_COUNT(index, step) ((index##Val0) + (step)*kCfiIncrement)
141 #define CFI_FUNC_COUNTER_INCREMENT(table, index, step) \
143 HARDENED_CHECK_EQ(table[index], CFI_STEP_TO_COUNT(index, step)); \
144 table[index] += kCfiIncrement; \
145 barrier32(table[index]); \
168 #define CFI_FUNC_COUNTER_PREPCALL(table, src, src_step, target) \
170 CFI_FUNC_COUNTER_INCREMENT(table, src, src_step); \
171 CFI_FUNC_COUNTER_INIT(table, target); \
172 CFI_FUNC_COUNTER_INCREMENT(table, src, (src_step + 1)); \
183 #define CFI_FUNC_COUNTER_CHECK(table, index, step) \
185 HARDENED_CHECK_EQ(table[index], CFI_STEP_TO_COUNT(index, step)); \