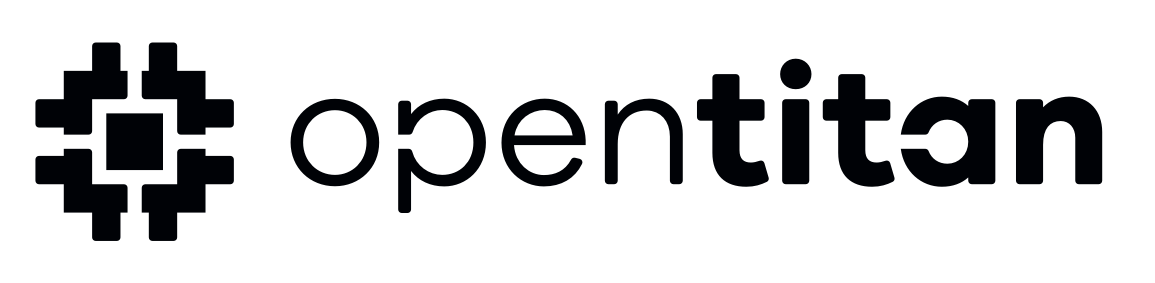 |
Software APIs
|
5 #ifndef OPENTITAN_SW_DEVICE_LIB_BASE_INTERNAL_STATUS_H_
6 #define OPENTITAN_SW_DEVICE_LIB_BASE_INTERNAL_STATUS_H_
8 #ifndef USING_INTERNAL_STATUS
9 #error "Do not include internal/status.h directly. Include status.h instead."
13 #define USING_ABSL_STATUS
14 #include "sw/device/lib/base/internal/absl_status.h"
15 #undef USING_ABSL_STATUS
50 #define STATUS_FIELD_CODE ((bitfield_field32_t){.mask = 0x1f, .index = 0})
51 #define STATUS_FIELD_ARG ((bitfield_field32_t){.mask = 0x7ff, .index = 5})
52 #define STATUS_FIELD_MODULE_ID \
53 ((bitfield_field32_t){.mask = 0x7fff, .index = 16})
54 #define STATUS_BIT_ERROR 31
57 #define ASCII_5BIT(v) ( \
58 (v) >= '@' && (v) <= '_' ? OT_UNSIGNED((v) - '@') \
59 : (v) >= '`' && (v) <= 'z' ? OT_UNSIGNED((v) - '`') \
60 : OT_UNSIGNED('_' - '@') \
76 #define MAKE_MODULE_ID(a, b, c) \
77 (ASCII_5BIT(a) << 16) | (ASCII_5BIT(b) << 21) | (ASCII_5BIT(c) << 26)