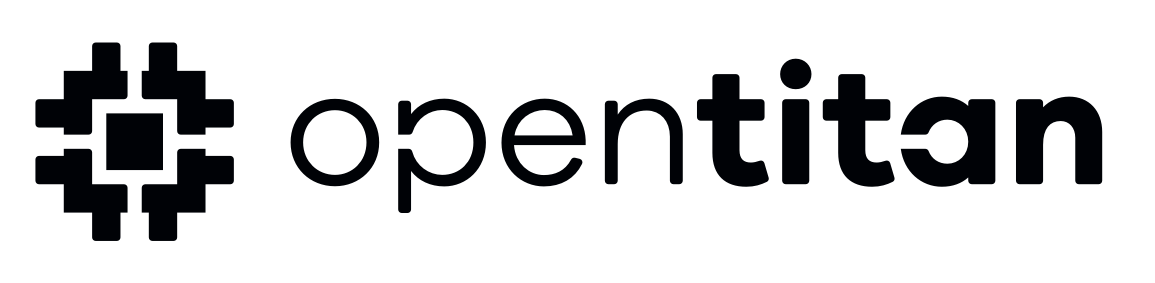 |
Software APIs
|
Go to the documentation of this file.
13#ifndef _PWRMGR_REG_DEFS_
14#define _PWRMGR_REG_DEFS_
20#define PWRMGR_PARAM_NUM_WKUPS 6
24#define PWRMGR_PARAM_SYSRST_CTRL_AON_WKUP_REQ_IDX 0
28#define PWRMGR_PARAM_ADC_CTRL_AON_WKUP_REQ_IDX 1
32#define PWRMGR_PARAM_PINMUX_AON_PIN_WKUP_REQ_IDX 2
36#define PWRMGR_PARAM_PINMUX_AON_USB_WKUP_REQ_IDX 3
40#define PWRMGR_PARAM_AON_TIMER_AON_WKUP_REQ_IDX 4
44#define PWRMGR_PARAM_SENSOR_CTRL_AON_WKUP_REQ_IDX 5
47#define PWRMGR_PARAM_NUM_RST_REQS 2
50#define PWRMGR_PARAM_NUM_INT_RST_REQS 2
53#define PWRMGR_PARAM_NUM_DEBUG_RST_REQS 1
56#define PWRMGR_PARAM_NUM_ROM_INPUTS 1
59#define PWRMGR_PARAM_RESET_MAIN_PWR_IDX 2
62#define PWRMGR_PARAM_RESET_ESC_IDX 3
65#define PWRMGR_PARAM_RESET_NDM_IDX 4
68#define PWRMGR_PARAM_NUM_ALERTS 1
71#define PWRMGR_PARAM_REG_WIDTH 32
74#define PWRMGR_INTR_COMMON_WAKEUP_BIT 0
77#define PWRMGR_INTR_STATE_REG_OFFSET 0x0
78#define PWRMGR_INTR_STATE_REG_RESVAL 0x0u
79#define PWRMGR_INTR_STATE_WAKEUP_BIT 0
82#define PWRMGR_INTR_ENABLE_REG_OFFSET 0x4
83#define PWRMGR_INTR_ENABLE_REG_RESVAL 0x0u
84#define PWRMGR_INTR_ENABLE_WAKEUP_BIT 0
87#define PWRMGR_INTR_TEST_REG_OFFSET 0x8
88#define PWRMGR_INTR_TEST_REG_RESVAL 0x0u
89#define PWRMGR_INTR_TEST_WAKEUP_BIT 0
92#define PWRMGR_ALERT_TEST_REG_OFFSET 0xc
93#define PWRMGR_ALERT_TEST_REG_RESVAL 0x0u
94#define PWRMGR_ALERT_TEST_FATAL_FAULT_BIT 0
97#define PWRMGR_CTRL_CFG_REGWEN_REG_OFFSET 0x10
98#define PWRMGR_CTRL_CFG_REGWEN_REG_RESVAL 0x1u
99#define PWRMGR_CTRL_CFG_REGWEN_EN_BIT 0
102#define PWRMGR_CONTROL_REG_OFFSET 0x14
103#define PWRMGR_CONTROL_REG_RESVAL 0x180u
104#define PWRMGR_CONTROL_LOW_POWER_HINT_BIT 0
105#define PWRMGR_CONTROL_LOW_POWER_HINT_VALUE_NONE 0x0
106#define PWRMGR_CONTROL_LOW_POWER_HINT_VALUE_LOW_POWER 0x1
107#define PWRMGR_CONTROL_CORE_CLK_EN_BIT 4
108#define PWRMGR_CONTROL_CORE_CLK_EN_VALUE_DISABLED 0x0
109#define PWRMGR_CONTROL_CORE_CLK_EN_VALUE_ENABLED 0x1
110#define PWRMGR_CONTROL_IO_CLK_EN_BIT 5
111#define PWRMGR_CONTROL_IO_CLK_EN_VALUE_DISABLED 0x0
112#define PWRMGR_CONTROL_IO_CLK_EN_VALUE_ENABLED 0x1
113#define PWRMGR_CONTROL_USB_CLK_EN_LP_BIT 6
114#define PWRMGR_CONTROL_USB_CLK_EN_LP_VALUE_DISABLED 0x0
115#define PWRMGR_CONTROL_USB_CLK_EN_LP_VALUE_ENABLED 0x1
116#define PWRMGR_CONTROL_USB_CLK_EN_ACTIVE_BIT 7
117#define PWRMGR_CONTROL_USB_CLK_EN_ACTIVE_VALUE_DISABLED 0x0
118#define PWRMGR_CONTROL_USB_CLK_EN_ACTIVE_VALUE_ENABLED 0x1
119#define PWRMGR_CONTROL_MAIN_PD_N_BIT 8
120#define PWRMGR_CONTROL_MAIN_PD_N_VALUE_POWER_DOWN 0x0
121#define PWRMGR_CONTROL_MAIN_PD_N_VALUE_POWER_UP 0x1
125#define PWRMGR_CFG_CDC_SYNC_REG_OFFSET 0x18
126#define PWRMGR_CFG_CDC_SYNC_REG_RESVAL 0x0u
127#define PWRMGR_CFG_CDC_SYNC_SYNC_BIT 0
130#define PWRMGR_WAKEUP_EN_REGWEN_REG_OFFSET 0x1c
131#define PWRMGR_WAKEUP_EN_REGWEN_REG_RESVAL 0x1u
132#define PWRMGR_WAKEUP_EN_REGWEN_EN_BIT 0
135#define PWRMGR_WAKEUP_EN_EN_FIELD_WIDTH 1
136#define PWRMGR_WAKEUP_EN_MULTIREG_COUNT 1
139#define PWRMGR_WAKEUP_EN_REG_OFFSET 0x20
140#define PWRMGR_WAKEUP_EN_REG_RESVAL 0x0u
141#define PWRMGR_WAKEUP_EN_EN_0_BIT 0
142#define PWRMGR_WAKEUP_EN_EN_1_BIT 1
143#define PWRMGR_WAKEUP_EN_EN_2_BIT 2
144#define PWRMGR_WAKEUP_EN_EN_3_BIT 3
145#define PWRMGR_WAKEUP_EN_EN_4_BIT 4
146#define PWRMGR_WAKEUP_EN_EN_5_BIT 5
150#define PWRMGR_WAKE_STATUS_VAL_FIELD_WIDTH 1
151#define PWRMGR_WAKE_STATUS_MULTIREG_COUNT 1
154#define PWRMGR_WAKE_STATUS_REG_OFFSET 0x24
155#define PWRMGR_WAKE_STATUS_REG_RESVAL 0x0u
156#define PWRMGR_WAKE_STATUS_VAL_0_BIT 0
157#define PWRMGR_WAKE_STATUS_VAL_1_BIT 1
158#define PWRMGR_WAKE_STATUS_VAL_2_BIT 2
159#define PWRMGR_WAKE_STATUS_VAL_3_BIT 3
160#define PWRMGR_WAKE_STATUS_VAL_4_BIT 4
161#define PWRMGR_WAKE_STATUS_VAL_5_BIT 5
164#define PWRMGR_RESET_EN_REGWEN_REG_OFFSET 0x28
165#define PWRMGR_RESET_EN_REGWEN_REG_RESVAL 0x1u
166#define PWRMGR_RESET_EN_REGWEN_EN_BIT 0
169#define PWRMGR_RESET_EN_EN_FIELD_WIDTH 1
170#define PWRMGR_RESET_EN_MULTIREG_COUNT 1
173#define PWRMGR_RESET_EN_REG_OFFSET 0x2c
174#define PWRMGR_RESET_EN_REG_RESVAL 0x0u
175#define PWRMGR_RESET_EN_EN_0_BIT 0
176#define PWRMGR_RESET_EN_EN_1_BIT 1
180#define PWRMGR_RESET_STATUS_VAL_FIELD_WIDTH 1
181#define PWRMGR_RESET_STATUS_MULTIREG_COUNT 1
184#define PWRMGR_RESET_STATUS_REG_OFFSET 0x30
185#define PWRMGR_RESET_STATUS_REG_RESVAL 0x0u
186#define PWRMGR_RESET_STATUS_VAL_0_BIT 0
187#define PWRMGR_RESET_STATUS_VAL_1_BIT 1
190#define PWRMGR_ESCALATE_RESET_STATUS_REG_OFFSET 0x34
191#define PWRMGR_ESCALATE_RESET_STATUS_REG_RESVAL 0x0u
192#define PWRMGR_ESCALATE_RESET_STATUS_VAL_BIT 0
195#define PWRMGR_WAKE_INFO_CAPTURE_DIS_REG_OFFSET 0x38
196#define PWRMGR_WAKE_INFO_CAPTURE_DIS_REG_RESVAL 0x0u
197#define PWRMGR_WAKE_INFO_CAPTURE_DIS_VAL_BIT 0
200#define PWRMGR_WAKE_INFO_REG_OFFSET 0x3c
201#define PWRMGR_WAKE_INFO_REG_RESVAL 0x0u
202#define PWRMGR_WAKE_INFO_REASONS_MASK 0x3fu
203#define PWRMGR_WAKE_INFO_REASONS_OFFSET 0
204#define PWRMGR_WAKE_INFO_REASONS_FIELD \
205 ((bitfield_field32_t) { .mask = PWRMGR_WAKE_INFO_REASONS_MASK, .index = PWRMGR_WAKE_INFO_REASONS_OFFSET })
206#define PWRMGR_WAKE_INFO_FALL_THROUGH_BIT 6
207#define PWRMGR_WAKE_INFO_ABORT_BIT 7
210#define PWRMGR_FAULT_STATUS_REG_OFFSET 0x40
211#define PWRMGR_FAULT_STATUS_REG_RESVAL 0x0u
212#define PWRMGR_FAULT_STATUS_REG_INTG_ERR_BIT 0
213#define PWRMGR_FAULT_STATUS_ESC_TIMEOUT_BIT 1
214#define PWRMGR_FAULT_STATUS_MAIN_PD_GLITCH_BIT 2