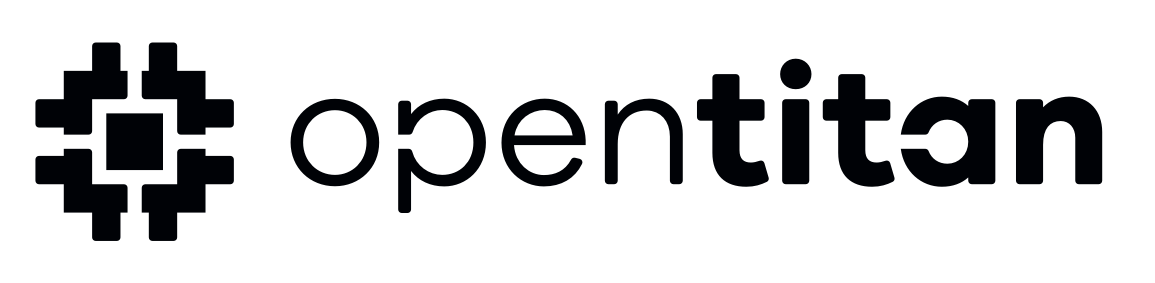 |
Software APIs
|
Go to the documentation of this file.
13#ifndef _PWRMGR_REG_DEFS_
14#define _PWRMGR_REG_DEFS_
20#define PWRMGR_PARAM_NUM_WKUPS 4
24#define PWRMGR_PARAM_PINMUX_AON_PIN_WKUP_REQ_IDX 0
28#define PWRMGR_PARAM_AON_TIMER_AON_WKUP_REQ_IDX 1
32#define PWRMGR_PARAM_SOC_PROXY_WKUP_INTERNAL_REQ_IDX 2
36#define PWRMGR_PARAM_SOC_PROXY_WKUP_EXTERNAL_REQ_IDX 3
39#define PWRMGR_PARAM_NUM_RST_REQS 2
42#define PWRMGR_PARAM_NUM_INT_RST_REQS 2
45#define PWRMGR_PARAM_NUM_DEBUG_RST_REQS 1
48#define PWRMGR_PARAM_NUM_ROM_INPUTS 3
51#define PWRMGR_PARAM_RESET_MAIN_PWR_IDX 2
54#define PWRMGR_PARAM_RESET_ESC_IDX 3
57#define PWRMGR_PARAM_RESET_NDM_IDX 4
60#define PWRMGR_PARAM_NUM_ALERTS 1
63#define PWRMGR_PARAM_REG_WIDTH 32
66#define PWRMGR_INTR_COMMON_WAKEUP_BIT 0
69#define PWRMGR_INTR_STATE_REG_OFFSET 0x0
70#define PWRMGR_INTR_STATE_REG_RESVAL 0x0u
71#define PWRMGR_INTR_STATE_WAKEUP_BIT 0
74#define PWRMGR_INTR_ENABLE_REG_OFFSET 0x4
75#define PWRMGR_INTR_ENABLE_REG_RESVAL 0x0u
76#define PWRMGR_INTR_ENABLE_WAKEUP_BIT 0
79#define PWRMGR_INTR_TEST_REG_OFFSET 0x8
80#define PWRMGR_INTR_TEST_REG_RESVAL 0x0u
81#define PWRMGR_INTR_TEST_WAKEUP_BIT 0
84#define PWRMGR_ALERT_TEST_REG_OFFSET 0xc
85#define PWRMGR_ALERT_TEST_REG_RESVAL 0x0u
86#define PWRMGR_ALERT_TEST_FATAL_FAULT_BIT 0
89#define PWRMGR_CTRL_CFG_REGWEN_REG_OFFSET 0x10
90#define PWRMGR_CTRL_CFG_REGWEN_REG_RESVAL 0x1u
91#define PWRMGR_CTRL_CFG_REGWEN_EN_BIT 0
94#define PWRMGR_CONTROL_REG_OFFSET 0x14
95#define PWRMGR_CONTROL_REG_RESVAL 0x40u
96#define PWRMGR_CONTROL_LOW_POWER_HINT_BIT 0
97#define PWRMGR_CONTROL_LOW_POWER_HINT_VALUE_NONE 0x0
98#define PWRMGR_CONTROL_LOW_POWER_HINT_VALUE_LOW_POWER 0x1
99#define PWRMGR_CONTROL_CORE_CLK_EN_BIT 4
100#define PWRMGR_CONTROL_CORE_CLK_EN_VALUE_DISABLED 0x0
101#define PWRMGR_CONTROL_CORE_CLK_EN_VALUE_ENABLED 0x1
102#define PWRMGR_CONTROL_IO_CLK_EN_BIT 5
103#define PWRMGR_CONTROL_IO_CLK_EN_VALUE_DISABLED 0x0
104#define PWRMGR_CONTROL_IO_CLK_EN_VALUE_ENABLED 0x1
105#define PWRMGR_CONTROL_MAIN_PD_N_BIT 6
106#define PWRMGR_CONTROL_MAIN_PD_N_VALUE_POWER_DOWN 0x0
107#define PWRMGR_CONTROL_MAIN_PD_N_VALUE_POWER_UP 0x1
111#define PWRMGR_CFG_CDC_SYNC_REG_OFFSET 0x18
112#define PWRMGR_CFG_CDC_SYNC_REG_RESVAL 0x0u
113#define PWRMGR_CFG_CDC_SYNC_SYNC_BIT 0
116#define PWRMGR_WAKEUP_EN_REGWEN_REG_OFFSET 0x1c
117#define PWRMGR_WAKEUP_EN_REGWEN_REG_RESVAL 0x1u
118#define PWRMGR_WAKEUP_EN_REGWEN_EN_BIT 0
121#define PWRMGR_WAKEUP_EN_EN_FIELD_WIDTH 1
122#define PWRMGR_WAKEUP_EN_MULTIREG_COUNT 1
125#define PWRMGR_WAKEUP_EN_REG_OFFSET 0x20
126#define PWRMGR_WAKEUP_EN_REG_RESVAL 0x0u
127#define PWRMGR_WAKEUP_EN_EN_0_BIT 0
128#define PWRMGR_WAKEUP_EN_EN_1_BIT 1
129#define PWRMGR_WAKEUP_EN_EN_2_BIT 2
130#define PWRMGR_WAKEUP_EN_EN_3_BIT 3
134#define PWRMGR_WAKE_STATUS_VAL_FIELD_WIDTH 1
135#define PWRMGR_WAKE_STATUS_MULTIREG_COUNT 1
138#define PWRMGR_WAKE_STATUS_REG_OFFSET 0x24
139#define PWRMGR_WAKE_STATUS_REG_RESVAL 0x0u
140#define PWRMGR_WAKE_STATUS_VAL_0_BIT 0
141#define PWRMGR_WAKE_STATUS_VAL_1_BIT 1
142#define PWRMGR_WAKE_STATUS_VAL_2_BIT 2
143#define PWRMGR_WAKE_STATUS_VAL_3_BIT 3
146#define PWRMGR_RESET_EN_REGWEN_REG_OFFSET 0x28
147#define PWRMGR_RESET_EN_REGWEN_REG_RESVAL 0x1u
148#define PWRMGR_RESET_EN_REGWEN_EN_BIT 0
151#define PWRMGR_RESET_EN_EN_FIELD_WIDTH 1
152#define PWRMGR_RESET_EN_MULTIREG_COUNT 1
155#define PWRMGR_RESET_EN_REG_OFFSET 0x2c
156#define PWRMGR_RESET_EN_REG_RESVAL 0x0u
157#define PWRMGR_RESET_EN_EN_0_BIT 0
158#define PWRMGR_RESET_EN_EN_1_BIT 1
162#define PWRMGR_RESET_STATUS_VAL_FIELD_WIDTH 1
163#define PWRMGR_RESET_STATUS_MULTIREG_COUNT 1
166#define PWRMGR_RESET_STATUS_REG_OFFSET 0x30
167#define PWRMGR_RESET_STATUS_REG_RESVAL 0x0u
168#define PWRMGR_RESET_STATUS_VAL_0_BIT 0
169#define PWRMGR_RESET_STATUS_VAL_1_BIT 1
172#define PWRMGR_ESCALATE_RESET_STATUS_REG_OFFSET 0x34
173#define PWRMGR_ESCALATE_RESET_STATUS_REG_RESVAL 0x0u
174#define PWRMGR_ESCALATE_RESET_STATUS_VAL_BIT 0
177#define PWRMGR_WAKE_INFO_CAPTURE_DIS_REG_OFFSET 0x38
178#define PWRMGR_WAKE_INFO_CAPTURE_DIS_REG_RESVAL 0x0u
179#define PWRMGR_WAKE_INFO_CAPTURE_DIS_VAL_BIT 0
182#define PWRMGR_WAKE_INFO_REG_OFFSET 0x3c
183#define PWRMGR_WAKE_INFO_REG_RESVAL 0x0u
184#define PWRMGR_WAKE_INFO_REASONS_MASK 0xfu
185#define PWRMGR_WAKE_INFO_REASONS_OFFSET 0
186#define PWRMGR_WAKE_INFO_REASONS_FIELD \
187 ((bitfield_field32_t) { .mask = PWRMGR_WAKE_INFO_REASONS_MASK, .index = PWRMGR_WAKE_INFO_REASONS_OFFSET })
188#define PWRMGR_WAKE_INFO_FALL_THROUGH_BIT 4
189#define PWRMGR_WAKE_INFO_ABORT_BIT 5
192#define PWRMGR_FAULT_STATUS_REG_OFFSET 0x40
193#define PWRMGR_FAULT_STATUS_REG_RESVAL 0x0u
194#define PWRMGR_FAULT_STATUS_REG_INTG_ERR_BIT 0
195#define PWRMGR_FAULT_STATUS_ESC_TIMEOUT_BIT 1
196#define PWRMGR_FAULT_STATUS_MAIN_PD_GLITCH_BIT 2